
Gesture Events for Mobile Websites
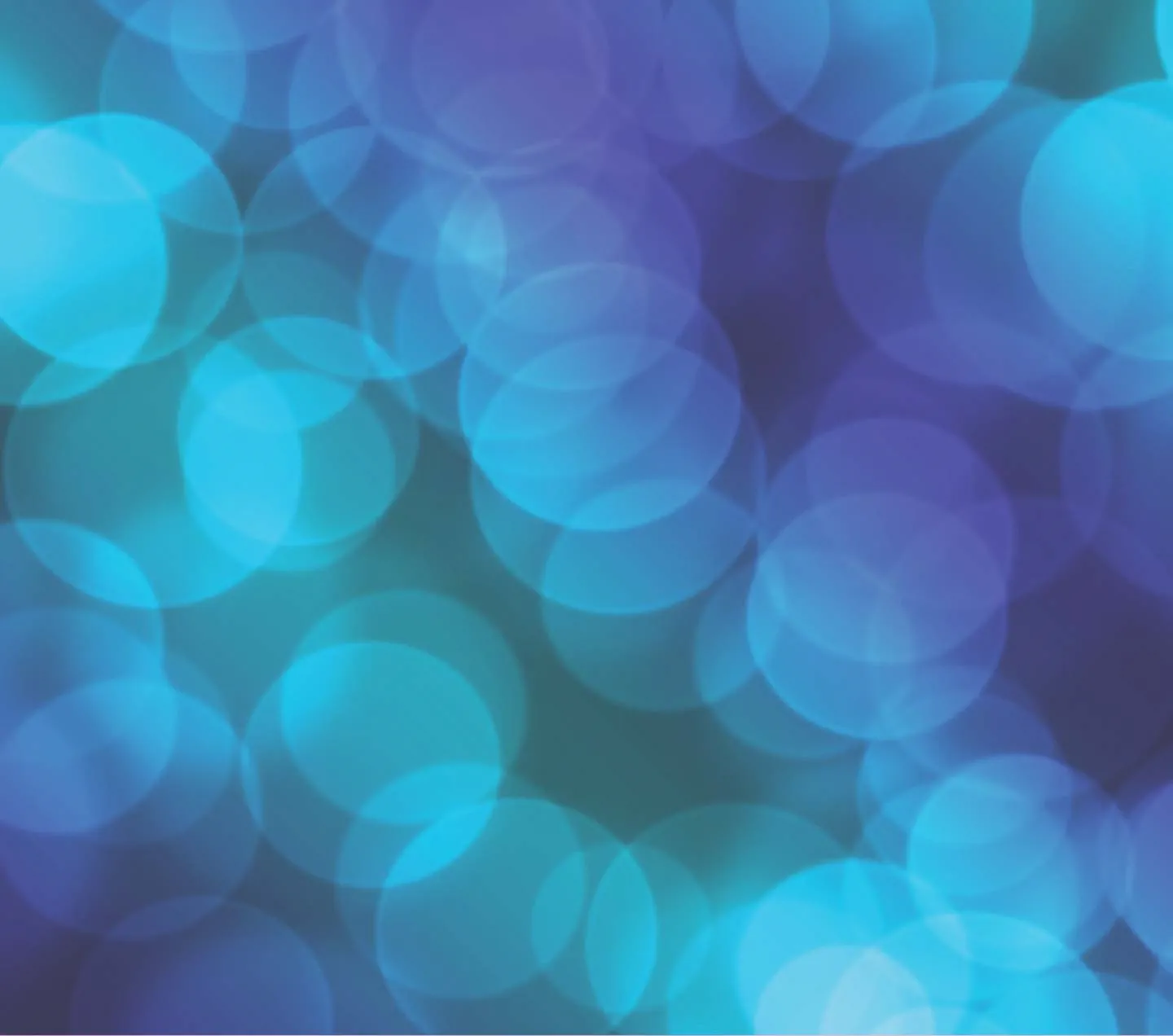


Nov 21, 2011
A requirement for every website is an easy and intuitive way for users to browse and navigate around a website. This requirement is also true when the user navigates in a mobile device, like a smart phone or tablet. Features like using a finger to swipe the content or using multi-touch gestures to zoom or rotate the content should be available on your site. These gestures can be modified to perform any different actions in a website.
In this article we will overwrite the default behavior for the pinch gesture to execute a “go back” function in an image gallery.
Gesture Events
Gesture events are events that are triggered during a multi-touch sequence, typically pinching and rotating gestures. Gesture events contain scaling and rotation information, allowing gestures to be combined.
Apple supports gestures that are multi-touch events such as “pinching” and “rotating”:
- gesturestart - triggered when initiating a multi-touch event
- gesturechange - triggered when multiple touches move
- gestureend - triggered when a multi-touch event ends/li>
The event object for gesture events looks very different. It contains scale and rotation values and no touch objects. The event object has the properties:
- event.scale - a value of 1 when there’s no scaling, less than 1 when zooming out (such as making our div smaller), and greater than 1 when zooming in.
- event.rotate - the rotation angle in degrees.
Note: The default behavior of Safari on iOS can interfere with your application’s custom multi-touch and gesture input. You can disable the default browser behavior by sending the preventDefault
message to the event object.
For more information on how to do this, visit this link
Example:
document.addEventListener('gesturechange', function(event) {
event.preventDefault();
console.log("Scale: " + event.scale + ", Rotation: " + event.rotation);
}, false);
Event Table
GESTURESTART | GESTUREMOVE | GESTUREEND | |
---|---|---|---|
Android | n | n | n |
iPhone | y | y | y |
has event.touches | n | n | n |
(iPhone) has event.scale and event.rotation | y | y | y |
(iPhone) touch in event.touches | - | - | - |
(iPhone) touch in event.changedTouches | - | - | - |
The code
To implement these functions we are going to use jQuery
First, we would need to include the jQuery library:
<!DOCTYPE html>
<html lang="es">
<head>
<script src="js/jquery.min.js" type="text/javascript"></script>
</head>
<body>
</body>
</html>
After that we will need to add the HTML structure:
<!DOCTYPE html>
<html lang="es">
<head>
<script src="js/jquery.min.js" type="text/javascript"></script>
</head>
<body>
<div style="width: 500px; margin: 0pt auto;">
<div>
<h1>click on an image</h1>
</div>
<div id="demos" class="content">
<div class="pics" id="slideshow">
<a href="#overlay01" ><img alt="" src="Images/logo001.png" /></a>
<a href="#overlay02" ><img alt="" src="Images/logo002.png" /></a>
<a href="#overlay03" ><img alt="" src="Images/logo003.png" /></a>
</div>
</div>
</div>
<div class="overlays" style="width: 500px; margin: 0pt auto;">
<div class="item" id="overlay01" >
<img alt="" src="Images/logo001.png" />
<p class="text">
pinch to close pinch to close pinch to close pinch to close
</p>
</div>
<div class="item" id="overlay02" >
<img alt="" src="Images/logo002.png" />
<p class="text">pinch to close pinch to close pinch to close pinch to close</p>
</div>
<div class="item" id="overlay03" >
<img alt="" src="Images/logo003.png" />
<p class="text">pinch to close pinch to close pinch to close pinch to close</p>
</div>
</div>
</body>
</html>
In the body structure we have a div with the class “pics” which holds the a set of images and a div with the class “overlays” which holds the overlay elements that are going to be shown when an image is clicked
Now we have to define the JavaScript code:
<script type="text/javascript">
$(function() {
$(".pics a").each(function(){
$(this).click(function(){
var overlay = $(this).attr('href');
$(overlay).show();
});
});
//bind events to swipe and pinch in overlay
$('.item').each(function(){
$(this).bind('gesturestart',function(){gestureStart(event);});
$(this).bind('gesturechange',function(){closeGesture(event);});
$(this).bind('gestureend',function(){gestureEnd(event);});
});
});
function gestureStart( e ) {
e.preventDefault();
}
function closeGesture( e ) {
e.preventDefault();
$('.item').hide();
}
function gestureEnd( e ) {
}
</script>
The JavaScript code will:
- Set up the click event for each image, with this code we are showing the corresponding overlay when the image is clicked:
$(".pics a").each(function(){
$(this).click(function(){
var overlay = $(this).attr('href');
$(overlay).show();
});
});
- Set up the event listener for each overlay, when zoom event is detected in an item overlay, we capture it and we change the default behavior with our custom functions:
$('.item').each(function(){
$(this).bind('gesturestart',function(){gestureStart(event);});
$(this).bind('gesturechange',function(){closeGesture(event);});
$(this).bind('gestureend',function(){gestureEnd(event);});
});
- Define our custom functions that are going to be executed in each step of the zoom events:
function gestureStart( e ) {
e.preventDefault();
}
function closeGesture( e ) {
e.preventDefault();
$('.item').hide();
}
function gestureEnd( e ) {
}
NOTE: that when the gesture starts we execute e.preventDefault();
this is done to prevent the default behavior of the browser (zoom in/out)
Using this method, we can detect the zoom in/out of an element of the web page and execute a custom code.
Recent Insights
-
-
Oshyn
Takeaways From Adobe Summit 2025
Agents, Creativity, and Vibrance
-
Fernando Torres
JotForm
A Comprehensive Guide
-