
Web Development Trends: Parallax Scrolling
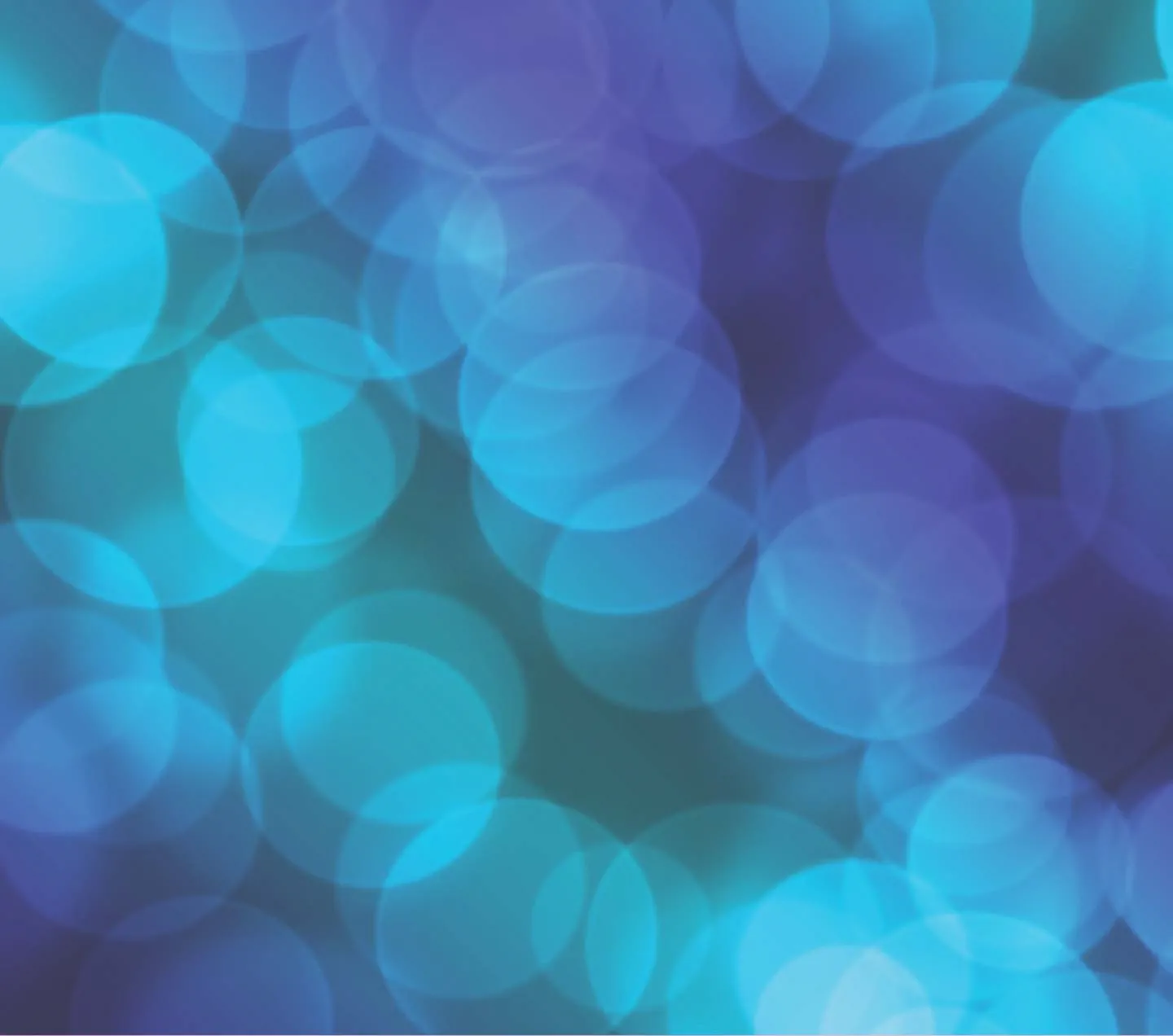


Mar 27, 2014
What is Parallax Scrolling?
Parallax scrolling is a special effect where background images move slower than foreground images, creating an illusion of depth. This technique was first popularized in video games.
The parallax effect became a trend in websites relatively recently and this cool effect is commonly part of the scrolling feature of a web page.
Examples of site using parallax
How to implement parallax scrolling with plugins
In addition to jQuery for this effect, we are going to use two more plugins to create a page with a depth effect and a scrolling feature.
-
jQuery.LocalScroll
This plugin will animate with a smooth scrolling effect and anchor navigation. jQuery.LocalScroll 1.2.x requires jQuery.ScrollTo 1.3.1 or higher.
-
jQuery.Scrolly
This plugin help us to create a depth effect. Scrolly is a super simple parallax plugin that can be applied to background images or directly to HTML elements.
We are going to implement a web page with three layers; each layer will have a different speed to create the effect of depth. The design concept of this example is to see the planet Earth from the moon. The stars layer, earth layer, and moon layer will have different speeds and in addition to that, we will set up a scrolling navigation. Follow these steps to create this parallax scrolling yourself:
Step 1.
Include the JavaScript libraries:
<script type="text/javascript" src="http://code.jquery.com/jquery-1.11.0.min.js"></script>
<script type="text/javascript" src="jquery.scrolly.js"></script>
<script type="text/javascript" src="jquery.localscroll-1.2.7-min.js"></script>
<script type="text/javascript" src="jquery.scrollTo-1.4.3.1-min.js"></script>
Step 2.
Create a HTML code for the scrolling navigation. The navigation will link to the specific parts of the page. With the LocalScroll plugin we will add a smooth scrolling effect for aesthetic purposes.
<ul id="nav">
<li><a href="#first" title="Next Section">link1</a></li>
<li><a href="#second" title="Next Section">link2</a></li>
<li><a href="#third" title="Next Section">link3</a></li>
</ul>
Step 3.
Create the HTML for the main section of the web page; this section will have the text and the main background image and, will be one of the layers that is going to move when the user scrolls down the page. This section will have two speeds: one for when the user scrolls the page, a very slow speed for the background, and one for the normal speed for the text.
<div id="main" data-velocity="-.1">
<div id="first">
<div class="story">
<div class="float-left">
<h2>Scroll Down</h2>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Praesent dui quam, dapibus sed erat sit amet, consectetur egestas sapien. Mauris a est libero. Morbi enim magna, congue at imperdiet ac, mattis sit amet mi. In vehicula venenatis ipsum ac eleifend. In malesuada elit vel nunc congue mollis. Quisque tincidunt semper sapien id egestas. Nunc enim quam, molestie eu fermentum at, interdum egestas tellus. Cras gravida felis nisi</p>
</div>
</div>
</div>
<div id="second">
<div class="story">
<div class="float-left">
<h2>Scroll Down</h2>
<p>Aliquam erat volutpat. Nunc iaculis magna quis mattis elementum. Curabitur a mollis libero. Nam enim neque, commodo sit amet feugiat quis, interdum quis neque. Duis id mi vel lectus placerat consequat. Nam ipsum orci, luctus a imperdiet at, egestas vel orci. Nunc nec erat consequat sem varius tempus vitae vitae justo. Sed ut pharetra urna. Duis a congue est. Duis venenatis, nulla et euismod semper, arcu magna imperdiet libero</p>
<p>Sed a enim vitae dui tempus egestas. Vestibulum ante ipsum primis in faucibus orci luctus et ultrices posuere cubilia Curae; Nulla at urna sit amet justo pellentesque semper. Interdum et malesuada fames ac ante ipsum primis in faucibus. Duis semper turpis nec lectus tempor consectetur. Sed rutrum ac risus non faucibus. Phasellus ac sem accumsan, malesuada lorem vel, volutpat nisi. Etiam molestie convallis dui, a molestie elit bibendum a.</p>
</div>
</div>
</div>
<div id="third">
<div class="story">
<div class="float-left">
<h2>End</h2>
<p>Pellentesque faucibus, quam non dapibus fringilla, enim arcu euismod erat, eu aliquam dolor diam eu eros. </p>
</div>
</div>
</div>
</div>
Step 4.
In this step we are going to create the HTML for the earth layer and moon layer.
Step 5.
Create the styles for the HTML elements.
body{margin: 0;min-width: 980px;padding: 0;background-color: #000;}
h2{color:#fff;font-weight: 500;font-style: normal;}
p{ color: #fff; }
img{ border: 0; }
#main { padding-top: 50px; position: relative; z-index: 10; height: 2300px; background: url(stars01.png) 50% 0 repeat fixed;}
#nav { list-style: none; position: fixed; right: 20px; z-index: 999;}
#nav li { margin: 0 0 15px 0; }
.earth {left: 65%;position: absolute;top: 1300px;z-index: 10;}
.earth img { width: 200px; height: 200px; }
.moon{z-index:11; position:relative;}
.moon img { width: 100%; }
.float-left { float: left; margin: 0 0 0 20px; }
.float-right { float: right; margin: 0 20px 0 0;}
#first { height: 1000px; margin: 0 auto; padding: 0;}
#second { height: 1000px; margin: 0 auto; overflow: hidden; padding: 0;}
#third{ padding: 0; }
.story { margin: 0 auto; min-width: 980px; overflow: auto; width: 980px; }
.story .float-left, .story .float-right { position: relative; width: 350px;}
Step 6.
Now that we have all the HTML and the CSS, we’ll need is to create the JavaScript to simulate the parallax effect and the scrolling behavior.
Now it’s time to see this parallax site live. On the head section, we are going to add the following jQuery code:
<script>
$( document ).ready(function() {
//scroll to plugin
$('#nav').localScroll(800);
//Scrolly plugin is used to move any object or backgrounds
//used to move background
$('#main').scrolly({bgParallax: true});
//used to move an element
$('.earth').scrolly();
});
</script>
Conclusion
Parallax and Scrolling effects are a growing trend across the Web. There are a lot of websites that you can look to get more inspiration. Today I have shown you how to do a relatively simple parallax scrolling website that you can play around with it and build upon to create a unique, fun, and interactive site.
Plugins and pages used
jQuery Scrolldeck Parallax Plugin Demo
Related Insights
-
-
-
-
Jonathan Saurez
Unit Testing Using AEM Mocks
Detecting Code Flaws Before They Cause Outages for Your Users
This site is protected by reCAPTCHA and the Google Privacy Policy and Terms of Service apply.