
Sitecore Digital Marketing System Part 2: Creating Conditions to compare custom values
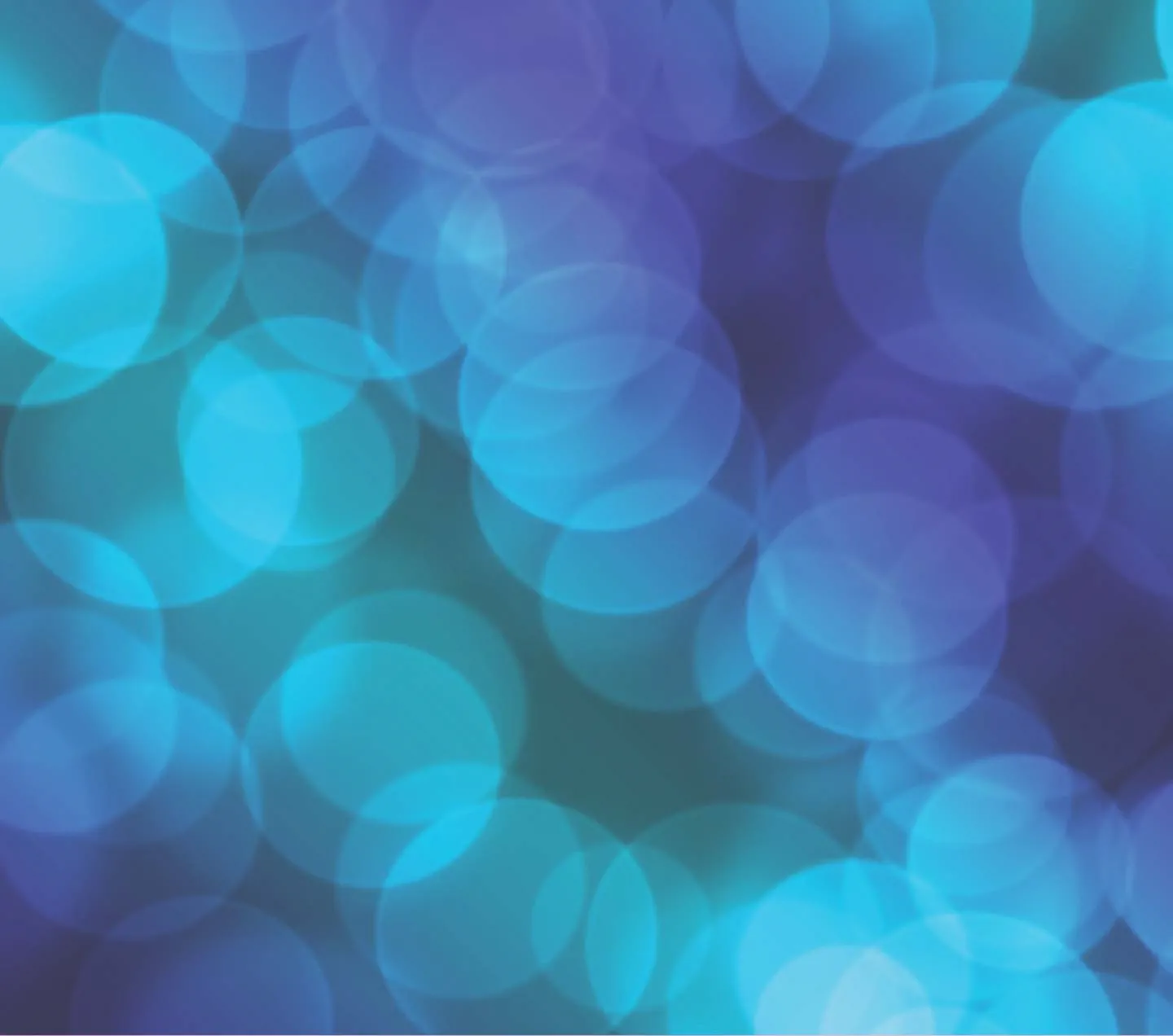


Apr 01, 2013
In part one of this post, I showed you how to create new conditions for your Sitecore Digital Marketing System site. Now that we know the process,I’ll add some sample code to create conditions that will compare integer, dates and custom values.
Integer Condition (Number of Achievements):
using Sitecore.Rules;
using Sitecore.Rules.Conditions;
namespace RulesEngine.Rules
{
public class AchievementsCondition<T> :
IntegerComparisonCondition<T> where T : RuleContext
{
//This is the value the editor will enter
//I prefer to have a separate string property otherwise I could
//use base.Value int property but there will be errors if
//the editor doesn't enter a number
public string Number { get; set; }
///
/// This function will be called to test the condition
///
protected override bool Execute(T ruleContext)
{
//Get the current user from our dummy user session manager
var user = UserSession.GetCurrentUser();
//Check if the user is logged in, otherwise return false
if (user == null)
return false;
int numberToCompare;
if (!Int32.TryParse(Number, out numberToCompare))
{
//Return false if the editor didn't enter an integer.
return false;
}
//Assign the value to base.Value to perform the comparison
Value = numberToCompare;
//Use base function to compare the values, this will compare
//base.Value with the parameter sent
return this.Compare(user.NumberAchievements);
}
}
}
For our condition’s text we will use the macro “Operator” instead of the StringOperator we used for the nationality condition because this one will allow us to compare numbers and not strings:
where the user's number of achievements [operatorid,Operator,,compares to] [Number,Text,,number]
Date Condition (Registration Date):
using Sitecore.Rules;
using Sitecore.Rules.Conditions;
namespace RulesEngine.Rules
{
public class RegistrationDateCondition<T> :
OperatorCondition<T> where T : RuleContext
{
//This is the value the editor will enter
public string StartDate { get; set; }
///
/// This function will be called to test the condition
///
protected override bool Execute(T ruleContext)
{
//Get the current user from our dummy user session manager
var user = UserSession.GetCurrentUser();
//Check if the user is logged in, otherwise return false
if (user == null)
return false;
//Check the editor entered a value
if (string.IsNullOrEmpty(StartDate))
{
return false;
}
DateTime userRegistrationDate = user.RegistrationDate;
DateTime startDate =
Sitecore.DateUtil.IsoDateToDateTime(StartDate);
//Do a date comparison and take the result return a value
var compareResult =
userRegistrationDate.Date.CompareTo(startDate.Date);
//Get the operator the editor selected
switch (this.GetOperator())
{
case ConditionOperator.Equal:
return compareResult == 0;
case ConditionOperator.GreaterThanOrEqual:
return compareResult == 0 || compareResult > 0;
case ConditionOperator.GreaterThan:
return compareResult > 0;
case ConditionOperator.LessThanOrEqual:
return compareResult == 0 || compareResult < 0;
case ConditionOperator.LessThan:
return compareResult < 0;
case ConditionOperator.NotEqual:
return compareResult != 0;
default:
return false;
}
}
}
}
For the condition text, we will use the macro “DateTime” because we need to select a date. Sitecore already has that functionality built in:
where user's registration date [operatorid,Operator,,compares to] [StartDate,DateTime,,date]
Custom Values Condition (Marital Status):
using Sitecore.Data.Items;
using Sitecore.Rules;
using Sitecore.Rules.Conditions;
namespace RulesEngine.Rules
{
public class MaritalStatusCondition :
WhenCondition where T : RuleContext
{
//This is the value the editor will pick
public string MaritalStatusId { get; set; }
///
/// This function will be called to test the condition
///
protected override bool Execute(T ruleContext)
{
//Get the current user from our dummy user session manager
var user = UserSession.GetCurrentUser();
//Check if the user is logged in, otherwise return false
if (user == null)
return false;
if (string.IsNullOrEmpty(MaritalStatusId))
return false;
//Read the item selected
Item maritalStatusItem =
Sitecore.Context.Database.GetItem(MaritalStatusId);
if (maritalStatusItem != null)
{
//To make this sample as simple as possible, I'm only going to
//use the the item's name
//Use base function to compare the values
return user.MaritalStatus
.ToString()
.Equals(maritalStatusItem.Name,
StringComparison.InvariantCultureIgnoreCase);
}
return false;
}
}
}
For the condition text, we will use “Tree” and send a parameter with the location of the list of items that the editor will pick from:
where the user matital status is [MaritalStatusId,Tree, root=/sitecore/content/Configuration/MaritalStatus, specific marital status]
Now we are able to select as many conditions as we need.
For more information about personalizing content for your site visitor, refer to the Rules Engine Cookbook.
Recent Insights
-
-
Oshyn
-
Oshyn
Takeaways From Adobe Summit 2025
Agents, Creativity, and Vibrance
-
Fernando Torres
JotForm
A Comprehensive Guide