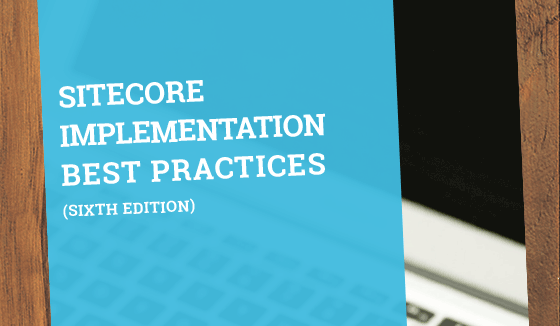
JavaScript Navigation using Hash Change "#"
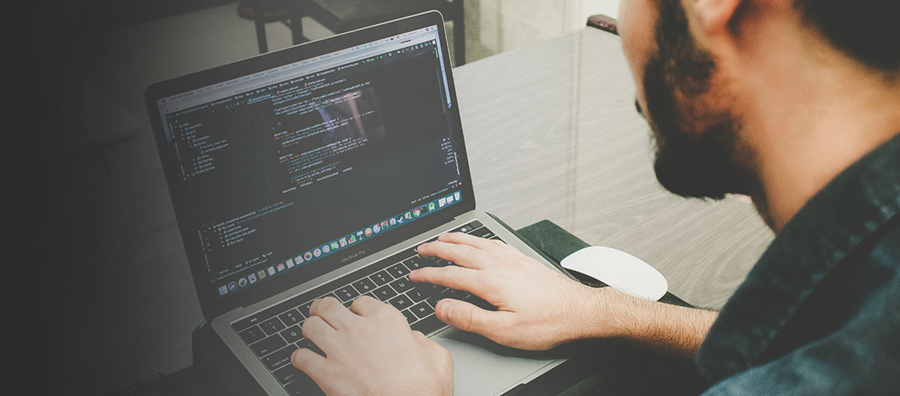


Dec 05, 2011
Modern web applications often load data or changes in the document object model (DOM) via AJAX without leaving the original page, to create a desktop-like experience. To do that we can take advantage of the URL hash value and the benefits it give us, including less bandwidth used by the client, faster response time and eventually a more interactive application feeling. By inserting a hash character into the URL we can pick up the string following it and use it as a value in AJAX requests. We can also create a listener event to check for a hash being added to a URL and use it to activate a custom JavaScript.
The hash symbol can enable backward and forward browser navigation support in a web page, without the need to reload the entire page. Using JavaScript and the “#” we can enable the browser history for Ajax calls and for DOM updates.
The content after the “#”in the URL is not sent to the server, since it has a hash in the URL. The content itself is accessible using JavaScript, using the variable window.location.hash. It has two big advantages when used with AJAX: first, it enables the browser history even if the web page is based on AJAX (this depends on the implementation, of course). Second, you can “share” or “bookmark” the link, so even if it is an Ajax call, it can be launched when you enter the page with the same link and with the same hash.
With JavaScript functions running and updating our DOM, we can change the hash with each JavaScript function. This means when the user hits the "back" button, the hash goes to the previous hash, and now since we have a listener, we can reload/re-render the previous content.
The most common form that uses the hash, and that many already know is to make a scroll down on the screen to the HTML tag with the ID assigned. For example:
If the hash in the URL indicates, for example: #end (http://www.domain.com/page.html#end
), the browser will scroll the screen to the item that has that ID.
Here, I will show you how to build a page that shows a set of images and when an image is clicked, it will show an overlay. With every event, the URL is going to change.
The Code
First, we would need to include the jQuery library:
<!DOCTYPE html>
<html lang="es">
<head>
<script src="js/jquery.min.js" type="text/javascript"></script>
</head>
<body>
</body>
</html>
After that we will need to add the HTML structure:
<!DOCTYPE html>
<html lang="es">
<head>
<script src="js/jquery.min.js" type="text/javascript"></script>
</head>
<body>
<div style="width: 500px; margin: 0pt auto;">
<div>
<h1>click on an image</h1>
</div>
<div id="demos" class="content">
<div class="pics" id="slideshow">
<a href="#overlay01" onclick="change_hash('overlay01'); return false; " ><img alt="" src="Images/logo001.png" /></a>
<a href="#overlay02" onclick="change_hash('overlay02'); return false; " ><img alt="" src="Images/logo002.png" /></a>
<a href="#overlay03" onclick="change_hash('overlay03'); return false; " ><img alt="" src="Images/logo003.png" /></a>
</div>
</div>
</div>
<div class="overlays" style="width: 500px; margin: 0pt auto;">
<div class="item" id="overlay01" >
<img alt="" src="Images/logo001.png" />
<p class="text">
click in the image to close overlay
</p>
</div>
<div class="item" id="overlay02" >
<img alt="" src="Images/logo002.png" />
<p class="text">
click in the image to close overlay
</p>
</div>
<div class="item" id="overlay03" >
<img alt="" src="Images/logo003.png" />
<p class="text">
click in the image to close overlay
</p>
</div>
</div>
</body>
</html>
In the body structure we have a div with the class “pics” which holds a set of images and a div with the class “overlays” which holds the overlay elements that are going to be shown when an image is clicked
Now we have to define the JavaScript code:
<script type="text/javascript">
$(function() {
//set up hash detection
$(window).bind( 'hashchange', function(e) {
var hash = '';
var hashlenght = 0;
hashwith = '';
if (location.hash == ''){
location.hash = '#pages=index';
document.title = 'Work Index';
}
hash = location.hash;
hashlenght = hash.length;
hashwith = '#'+hash.substring(7, hashlenght);
hash = location.hash.substring(7, hashlenght);
if(hashwith != "#"+'index'){
show_overlay(hash);
document.title = 'Work '+hash;
}
else{
close_overlay();
}
});
$(window).trigger( 'hashchange' );
$(".overlays .item img").each(function(){
$(this).click(function(){
close_overlay();
});
});
});
function close_overlay(){
$(".overlays .item").each(function(){
if($(this).css('display') == 'block'){$(this).hide();}
});
location.hash = '#pages=index';
}
//function to show overlay
function show_overlay(aux){
$("#"+aux).show();
}
//function to change hash
function change_hash(aux){
location.hash = '#pages='+aux;
document.title = 'Work '+aux;
}
</script>
The JavaScript code will:
- Bind a handler to the window.onhashchange event. Each time the hash changes is compared to the hash string to check if the page is in its original state or if an overlay was clicked
- The code receives the hash string to check if an overlay was clicked in the page, to execute a “show_overlay()” or “close_overlay()” function.
//set up hash detection
$(window).bind( 'hashchange', function(e) {
var hash = '';
var hashlenght = 0;
hashwith = '';
if (location.hash == ''){
location.hash = '#pages=index';
document.title = 'Work Index';
}
hash = location.hash;
hashlenght = hash.length;
hashwith = '#'+hash.substring(7, hashlenght);
hash = location.hash.substring(7, hashlenght);
if(hashwith != "#"+'index'){
show_overlay(hash);
document.title = 'Work '+hash;
}
else{
close_overlay();
}
});
- Trigger all bound window.onhashchange event handlers
$(window).trigger( 'hashchange' );
- Assign a function to close an opened overlay when the image in the overlay is clicked
$(".overlays .item img").each(function(){
$(this).click(function(){
close_overlay();
});
});
- Function to close an opened overlay
function close_overlay(){
$(".overlays .item").each(function(){
if($(this).css('display') == 'block'){$(this).hide();}
});
location.hash = '#pages=index';
}
- Function to show an overlay
//function to show overlay
function show_overlay(aux){
$("#"+aux).show();
}
- Function to change the hash
//function to change hash
function change_hash(aux){
location.hash = '#pages='+aux;
document.title = 'Work '+aux;
}
Although the hash can have powerful uses, it should not be the replacement of basic friendly URLs and permalinks that we all know. Google does not take into account the hash when indexing a page, the following pages will appear the same to Google and other search engines, and will not help with indexing and SEO:
- http://www.example.com /seccion/#Com45
- http://www.example.com /seccion/#445532q
- http://www.example.com /seccion/#file.html
Related Insights
This site is protected by reCAPTCHA and the Google Privacy Policy and Terms of Service apply.