
Creating Templates and Items in Sitecore by coding
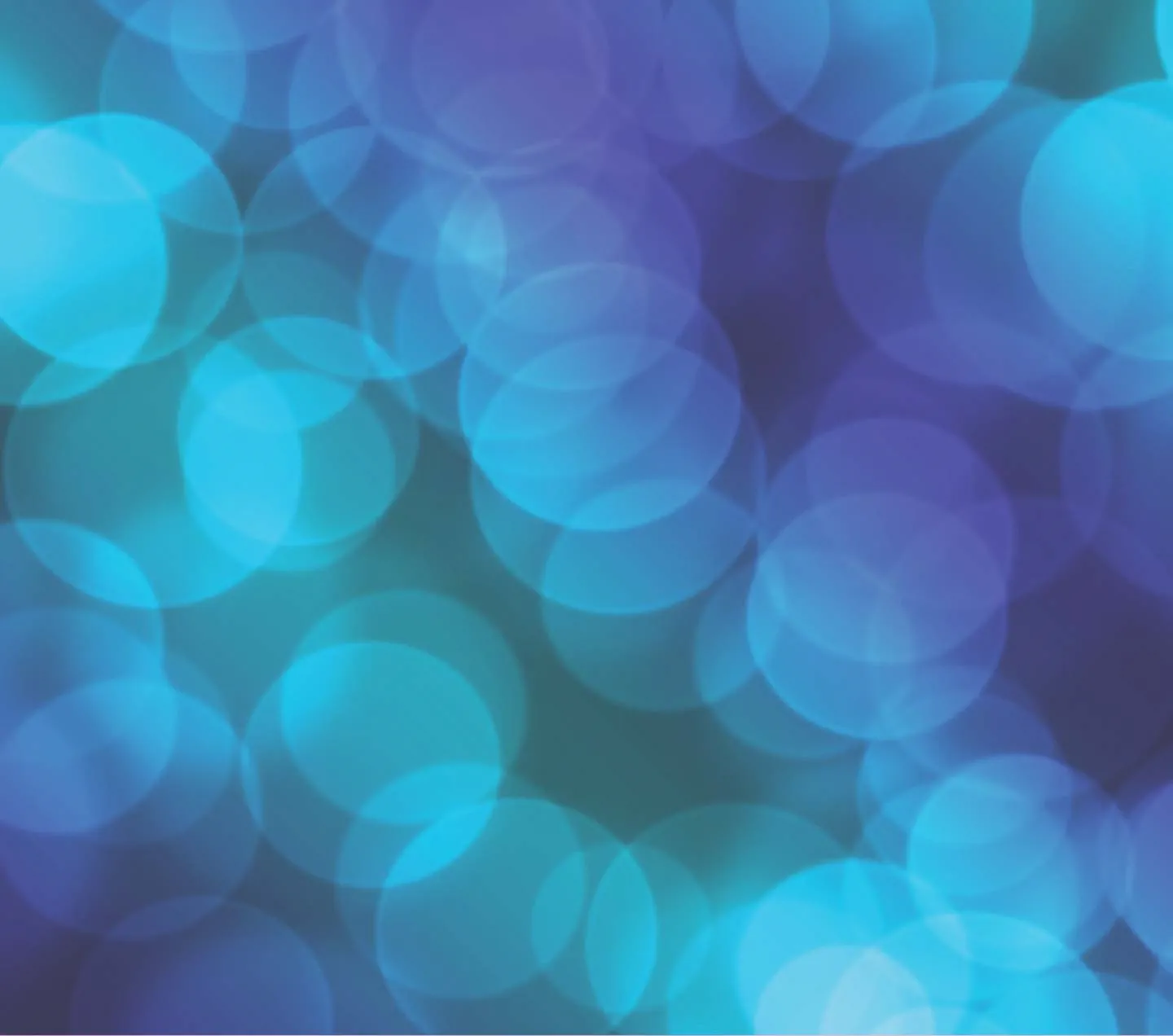


Jun 25, 2012
If we have looked around in Sitecore it is clear the idea of templates and items. As it says on the application manual, Sitecore treats everything as a template which is equals to a class in an object oriented language, and the items which are an instance of the class. This concept helps programmers to build organized components. But, this does not end here, programmatically Sitecore also maintains this concept and offers the chance to create and modify templates and items just by using its libraries in .Net.
This ability to create the site components and its instances by scripts in c# brings us the possibility to extend the functionalities of the site in different manners, like creating migration tools or custom tools on the Sitecore CMS. Knowing about these benefits in the following blog we present a brief detail on how to create this two major Sitecore components, templates and items.
In the picture above the home item is selected, on the right side description we can see the details of this item. First, we see the item has a path “/sitecore/content/Home”, and then we can see the template (class) of the item which is “/sitecore/templates/Sample/Sample Item”. This is the format how Sitecore works. Programmatically, we can see this structure as follows:
The first step is to use the Sitecore.Data.Items library.
Then we will look for the item and template on the database:
//To be able to access the information without restrictions we use the Sitecore.SecurityModel.SecurityDisabler
using (new Sitecore.SecurityModel.SecurityDisabler())
{
//Set the master database we will be working with.
Sitecore.Data.Database master = Sitecore.Configuration.Factory.GetDatabase("master");
//Look for the item in the database
Sitecore.Data.Items.Item item = master.Items.GetItem(“/sitecore/content/Home”);
//Get the template of the current item
Sitecore.Data.Items.TemplateItem template = item.Template;
}
With these steps it is possible to look for items and its properties.
However, programmatically the main process and functionality people use in Sitecore is to create templates and items, this is the base work a back end user will do to personalize or create tools.
To create a temple we will do the following: look if the template exists, if not create a template.
In the following example we will create a template under the User Defined folder
using (new Sitecore.SecurityModel.SecurityDisabler())
{
//Set the master database we will be working with.
Sitecore.Data.Database master = Sitecore.Configuration.Factory.GetDatabase(“master”);
//Look for the template on the database, if not found a null value will be set
Sitecore.Data.Items.TemplateItem templateItem = master.Templates[“/sitecore/Templates/User Defined/MyTemplate”];
//Check if template Item does not exists
if (templateItem == null)
{
//Get the parent Item of the template (folder)
Sitecore.Data.Items.Item parentItem = master.Items.GetItem(“/sitecore/Templates/User Defined”);
//Create the template under the given parent Item
templateItem = p_Database.Templates.CreateTemplate(“/sitecore/Templates/User Defined/MyTemplate”, parentItem);
}
}
In this code sample we can see that we look for the template we want to create first. Then if not found we look for the parent item. In this example the parent item is an item whose template is a folder. Then we create the template giving the template path and the parent item (folder).
Once we have created the template we can instantiate it as an item.
using (new Sitecore.SecurityModel.SecurityDisabler())
{
//Set the master database we will be working with.
Sitecore.Data.Database master = Sitecore.Configuration.Factory.GetDatabase("master");
//Look for the Item to check if exists
Sitecore.Data.Items.Item item = master.Items.GetItem(“/sitecore/content/Home/MyItem”);
if (item == null)
{
//Look for the TemplateItem we will be instantiating
Sitecore.Data.Items.TemplateItem templateItem = master.Templates[“/sitecore/Templates/User Defined/MyTemplate”];
//Create the Item based on the given template item
Item = p_Database.CreateItemPath(“/sitecore/content/Home/MyItem”, templateItem);
}
As a result of all this code, we have gotten the basic steps to look for a template and item and create each one of them.