
Coveo Search API from within Sitecore
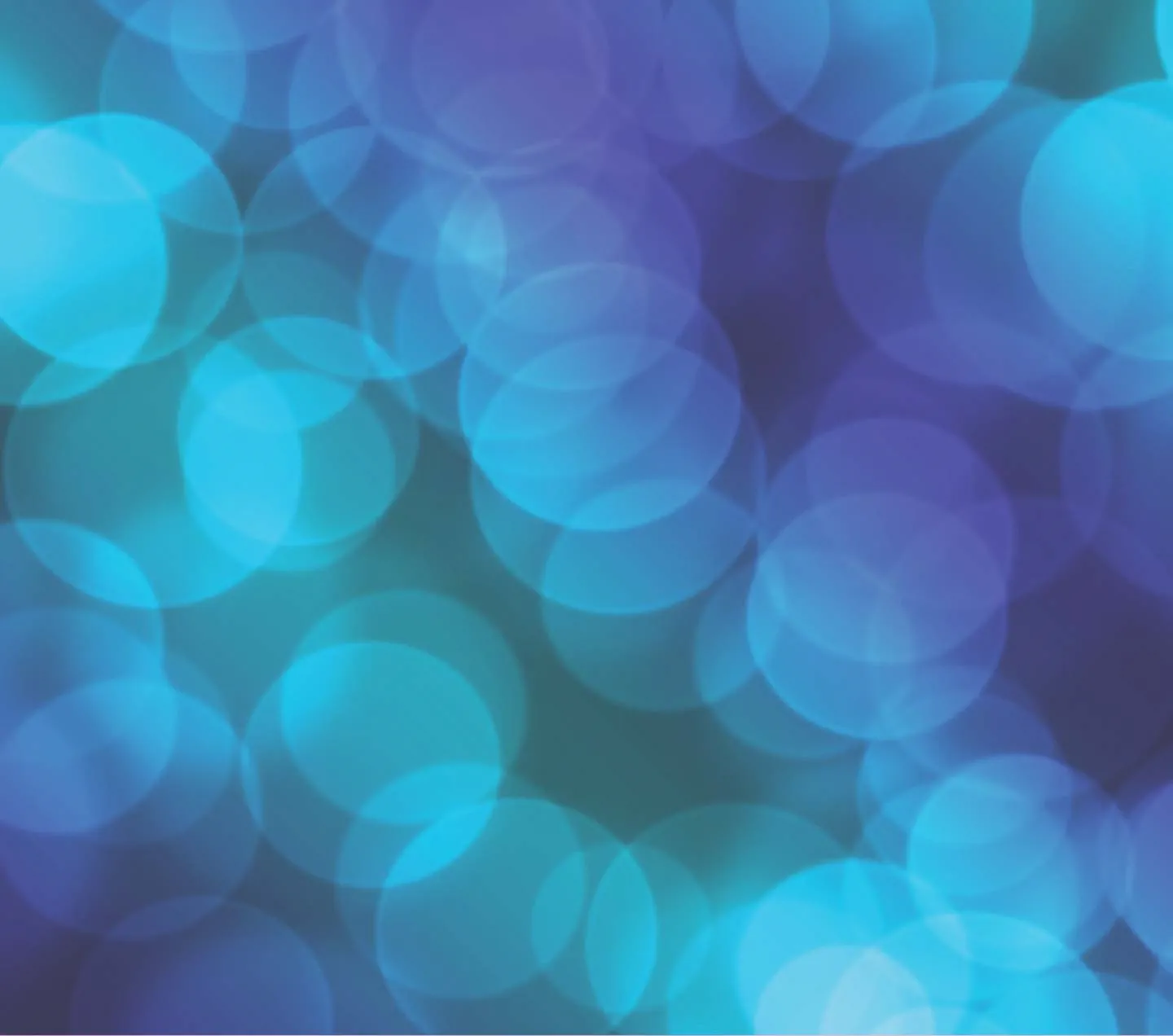


Jun 25, 2012
Coveo Enterprise Search for Sitecore has built-in functionality that allows you to search content and display the results on your website, and as I have explained in a previous post, it is also possible to change the style and the way search query and search results are shown; but sometimes this customization is not enough and it is needed to have more control in the query and how the results are shown.
Open your Visual Studio project and add a reference to Coveo.CES.Web.Search
and Coveo.CNL
.
We will use the following web form:

With this code:
<asp:TextBox ID=“txtTitle” runat=“server”></asp:TextBox>
<asp:Button ID=“SearchButton” runat=“server” Text=“Search” onclick=“SearchButton_Click” />
<br />
<br />
<asp:GridView ID=“resultsGrid” runat=“server” AutoGenerateColumns=“False”>
<Columns>
<asp:BoundField DataField=“SitecoreId” DataFormatString=“{{{0}}}” HeaderText=“Sitecore Id” />
<asp:BoundField DataField=“Title” HeaderText=“Title” />
<asp:BoundField DataField=“Author” HeaderText=“Author” />
</Columns>
<EmptyDataTemplate>
The query returned no results.
</EmptyDataTemplate>
</asp:GridView>
<asp:Label ID=“lblMessage” runat=“server” Text=“” EnableViewState=“false”></asp:Label>
And this code behind:
private void PerformSearch()
{
//Create the search builder object
SearchBuilder searchBuilder = CreateSearchBuilder();
//We will need 10 results
const int numberResults = 10;
//Start with the first item, this is used when paging the results
const int firstResult = 0;
//Sort the results by a field, ascending
searchBuilder.SortBy = Coveo.CES.Web.Search.SortByEnum.FieldAscending;
//Sort by the custom field @MyAuthor
searchBuilder.SortByField = "@MyAuthor";
//Search by the Title (@systitle)
searchBuilder.AddAdvancedExpression(string.Format("@systitle={0}", this.txtTitle.Text));
//Create query wrapper
QueryWrapper query = QueryWrapperFactory.GetNewQueryWrapper(searchBuilder);
//Perform the search
ICESResult[] searchResults = query.GetResults(firstResult, numberResults);
//Check if there are results
if (searchResults != null && searchResults.Length > 0)
{
//For this example, we will create an Anonymous Type (just to
//avoid the creation of an object entity)
//and assign the list to the GridView
var resultList = from result in searchResults
select new
{
Title = result.Title,
SitecoreId = result.GetField("@SCItemID").ToString(),
Author = result.GetField("@MyAuthor").ToString()
};
this.resultsGrid.DataSource = resultList;
this.resultsGrid.DataBind();
this.lblMessage.Text = string.Format("Showing {0} of {1} results", resultList.Count(), query.TotalCount);
}
else
{
this.resultsGrid.DataSource = null;
this.resultsGrid.DataBind();
}
}
private static SearchBuilder CreateSearchBuilder()
{
try
{
var builder = new SearchBuilder();
ICESSearchProvider provider = SearchProviderFactory.CreateDefaultSearchProvider();
builder.Provider = provider;
return builder;
}
catch (Exception ex)
{
//Log the error
return null;
}
}
Don’t forget to add the using statements:
using Coveo.CES.Web.Search.Providers;
using Coveo.CES.Web.Search.Controls;
This is the result:

In the next part of this post, we will do the same query via web services.
Now that we have learned how to query Coveo Enterprise Search by using Coveo DLLs from a Sitecore CMS page, I will show how to do the same query via web services; this code might even be useful for a desktop or mobile component of your Sitecore solution. Open your Visual Studio project and add a Service Reference to http://mycoveoserver:8080/CoveoSearch.asmx
We will use the same web form from the previous post:
With the same code:
<asp:TextBox ID=“txtTitle” runat=“server”></asp:TextBox>
<asp:Button ID=“SearchButton” runat=“server” Text=“Search” onclick=“SearchButton_Click” />
<br />
<br />
<asp:GridView ID=“resultsGrid” runat=“server” AutoGenerateColumns=“False”>
<Columns>
<asp:BoundField DataField=“SitecoreId” DataFormatString=“{{{0}}}” HeaderText=“Sitecore Id” />
<asp:BoundField DataField=“Title” HeaderText=“Title” />
<asp:BoundField DataField=“Author” HeaderText=“Author” />
</Columns>
<EmptyDataTemplate>
The query returned no results.
</EmptyDataTemplate>
</asp:GridView>
<asp:Label ID=“lblMessage” runat=“server” Text=“” EnableViewState=“false”></asp:Label>
And this code behind:
private void PerformSearch()
{
SearchServiceSoapClient searchService = new SearchServiceSoapClient();
//Create the query parameters object
QueryParameters queryParams = new QueryParameters();
//It is necessary to specify all the fields that are needed as results
ArrayOfString neededFields = new ArrayOfString();
//@SCItemID is the Sitecore Item ID
neededFields.Add(“@SCItemID”);
//We will need a custom field too
neededFields.Add(“@MyAuthor”);
//Assign the needed fields array
queryParams.NeededFields = neededFields;
//We will need 10 results
queryParams.NumberOfResults = 10;
//Start with the first item, this is used when paging the results
queryParams.FirstResult = 0;
//Sort the results by a field, ascending
queryParams.SortCriteria = SortByEnum.FieldAscending;
//Sort by the custom field @MyAuthor
queryParams.SortByField = “@MyAuthor”;
//Search by the Title (@systitle)
queryParams.AdvancedQuery = string.Format(“@systitle={0}”, this.txtTitle.Text);
//Perform the search
QueryResults searchResults = searchService.PerformQuery(queryParams);
//Check if there are results
if (searchResults != null &&
searchResults.Results != null &&
searchResults.Results.Length > 0)
{
//The results will be stored in the array ‘searchResults.Results’
//For this example, we will create an Anonymous Type (just to avoid the creation of an object entity)
//and assign the list to the GridView
var resultList = from result in searchResults.Results
select new
{
Title = result.Title,
SitecoreId = result.Fields[0].Value.ToString(),
Author = result.Fields[1].Value.ToString()
};
this.resultsGrid.DataSource = resultList;
this.resultsGrid.DataBind();
this.lblMessage.Text = string.Format(“Showing {0} of {1} results”, resultList.Count(), searchResults.TotalCount);
}
else
{
this.resultsGrid.DataSource = null;
this.resultsGrid.DataBind();
}
}
And we get the exact same results as we got in the previous post:
Recent Insights
-
-
Oshyn
Takeaways From Adobe Summit 2025
Agents, Creativity, and Vibrance
-
Fernando Torres
JotForm
A Comprehensive Guide
-